Laravel, a robust PHP framework, offers an elegant Object-Relational Mapping (ORM) called Eloquent, which simplifies interaction with databases. Eloquent provides an active record implementation for working with your database, allowing developers to interact with database objects and relationships using expressive syntax. By leveraging the power of model joins, Laravel enables fluid data management and query optimization. Understanding how to implement these features is crucial for building efficient and scalable applications. Keep reading to master the art of Eloquent relationships and model joins that can transform your Laravel projects.
Understanding Laravel’s Eloquent Relationships and Model Join
Laravel’s Eloquent ORM simplifies model relationships, like a blog post having many comments, using intuitive methods for associating different models. This ease extends to handling large datasets, where Laravel’s query builder makes it straightforward to execute complex queries, including performing a Laravel model join for fluid chaining and data retrieval.
The real power of Eloquent lies in its seamless integration with Laravel’s collection methods, which abstracts the complexity of SQL joins. By encouraging practices like eager loading to avoid the N + 1 query problem, Eloquent ensures optimized performance and cleaner, more readable code for developers.
Harnessing Eloquent’s Query Builder for Complex Joins
Laravel’s Eloquent ORM offers a powerful query builder for creating complex joins and data relations. Its expressive syntax allows developers to define data relationships without writing raw SQL queries. One notable feature is the ability to add constraints to joins, filtering data at the query level, enabling efficient data management and high performance.
The query builder can handle complex scenarios like joining with subqueries, making it both flexible and comprehensive. It also works well with Eloquent’s soft deletes and global scopes, allowing developers to include or exclude soft-deleted records in their joins, ensuring consistency across all database operations. This higher level of abstraction enhances the power of Laravel’s ORM for data manipulation.
Key Differences Between Relation Methods: has, whereHas, with, and join
Eloquent relation methods in Laravel offer various ways to access and manipulate related models. Understanding these methods is crucial for proper database querying. The ‘has’ method filters results based on the existence of related models, while ‘whereHas’ adds custom constraints to a relationship query. The ‘with’ method loads relationships efficiently, while ‘join’ allows developers to directly join tables. Each method has specific use cases and can enhance data retrieval efficiency.
It’s important to recognize the impact of these methods on query performance. ‘join’ can lead to faster queries but can complicate data retrieval when relationships become complex. ‘with’ can optimize performance by preemptively loading data, but may lead to unwanted data if not used carefully. Combining these methods can lead to more efficient and readable code.
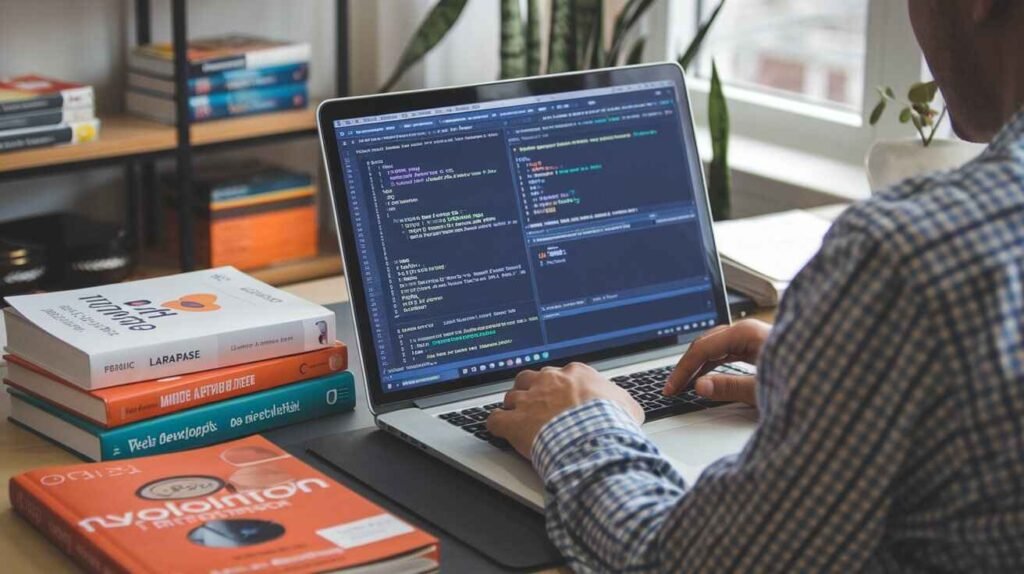
Best Practices for Optimizing Query Performance with Joins in Laravel
Laravel’s ecosystem offers a method for optimizing query performance with joins. Developers should structure queries to minimize database load, indexing foreign keys and frequently queried fields to speed up join operations. Eloquent models can also be structured carefully to avoid redundant queries and overhead. Laravel’s caching mechanisms can store complex join results, reducing the need to execute the same queries repeatedly.
For polymorphic relations or many-to-many joins, pagination and batch processing are crucial to process only a manageable subset of data at a time. Offloading read-intensive operations to a database replica can balance load and reduce latency in data retrieval, improving overall application speed and efficiency.
Advanced Techniques: Polymorphic and Many-to-Many Joins in Eloquent Models
Laravel’s ORM offers advanced Eloquent relationship patterns, such as polymorphic and many-to-many joins, which enable models to belong to multiple models on a single association. These relationships provide cleaner and more comprehensible models, especially when traditional relationships may not suffice. Many-to-many relationships, facilitated through pivot tables, add complexity but also flexibility to Eloquent models, especially when multiple entities share connections.
Laravel’s ORM handles this complexity, allowing for fluent data interactions. Developers can also use custom polymorphic types and pivot model events to customize the ORM’s capabilities to their application needs. Understanding the trade-offs of these relationships is crucial, as they may introduce additional queries and join statements that could impact performance. Careful consideration and rigorous testing are essential to ensure these advanced relationships enhance application performance.
Overall, the Laravel framework’s ORM presents a blend of simplicity and sophistication that empowers developers to construct complex data relationships with confidence. Harnessing the power of model joins, and understanding when and how to use various relationship methods, can significantly minimize queries and maximize application performance. A well-implemented use of joins within Eloquent can define the foundation of an intuitive and efficient database interaction layer.